pom配置
<dependencies> <dependency> <groupId>org.springframework.security.oauth</groupId> <artifactId>spring-security-oauth2</artifactId> <version>2.0.9.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> </dependencies>
security配置
水电费 @Configuration @EnableWebSecurity public class WebSecurityConfig extends WebSecurityConfigurerAdapter { @Override public void configure(HttpSecurity http) throws Exception { http.authorizeRequests() .antMatchers("/oauth/**").permitAll() .and() .csrf().disable(); } @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { // 基于内存的认证 auth.inMemoryAuthentication() .withUser("user") .password(new BCryptPasswordEncoder().encode("111111")) .roles("USER") .and() .withUser("admin") .password(new BCryptPasswordEncoder().encode("111111")) .roles("ADMIN"); } /** * 需要配置这个支持password模式 * support password grant type * @return * @throws Exception */ @Override @Bean public AuthenticationManager authenticationManagerBean() throws Exception { return super.authenticationManagerBean(); }
auth配置
@Configuration @EnableAuthorizationServer public class AuthorizationConfig extends AuthorizationServerConfigurerAdapter {@Autowired
private TokenStore tokenStore;
/**
* 注入authenticationManager
* 来支持 password grant type
*/
@Autowired
private AuthenticationManager authenticationManager;
/**
* 配置编码器
*/
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory() // 基于内存
.withClient("app") //授权客户端
.secret(passwordEncoder().encode("111111")) //授权码
.accessTokenValiditySeconds((int) TimeUnit.SECONDS.toSeconds(30)) // 授权过期时间
.authorizedGrantTypes("password", "refresh_token", "client_credentials") // 授权模式
.scopes("all") // 授权范围
.resourceIds("rid"); // 授权资源
}
@Override
public void configure(AuthorizationServerSecurityConfigurer oauthServer) throws Exception {
oauthServer .tokenKeyAccess("permitAll()")
.checkTokenAccess("permitAll()")
.allowFormAuthenticationForClients();
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenStore(tokenStore)
.authenticationManager(authenticationManager);
}
@Bean
public TokenStore tokenStore() {
//这里为了简单达到目的,直接使用内存存储Token和用户信息。
return new InMemoryTokenStore();
}
}
@EnableResourceServer @Configuration public class ResourceConfigure extends ResourceServerConfigurerAdapter {@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.resourceId("rid");
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/user/**").authenticated()// 只是告知授权才能访问
.antMatchers("/test/**").hasRole("ADMIN");// 当密码授权访问时,告知哪个角色能访问
}
}
测试Controller
@Slf4j @RestController @RequestMapping("/test") public class TestController {@RequestMapping("/hello")
public String hello() {
try {
return "Hello Word test";
} catch (Exception e) {
log.error("", e);
return "test/hello 异常";
}
}
}
@Slf4j @RestController @RequestMapping("/user") public class UserController {@RequestMapping("/hello")
public String hello() {
try {
return "Hello Word user";
} catch (Exception e) {
log.error("", e);
return "
user/hello 异常
";}
}
}
请求测试
password授权模式获取token
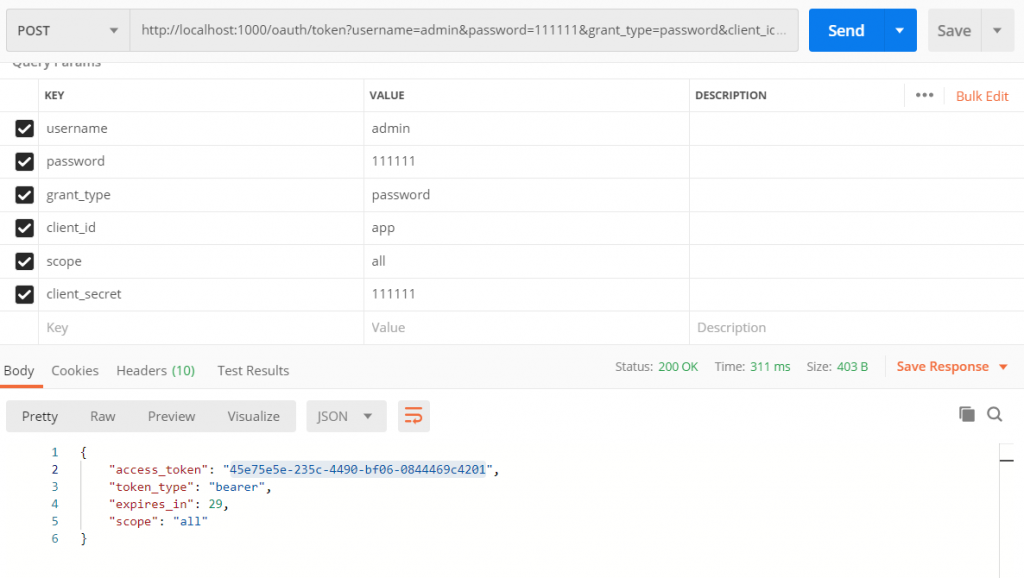
根据token访问后台
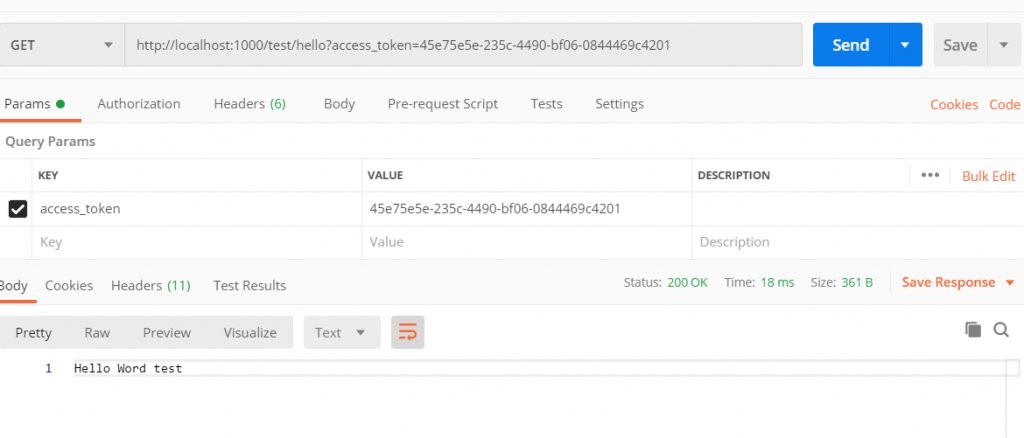
客户端授权模式获取token
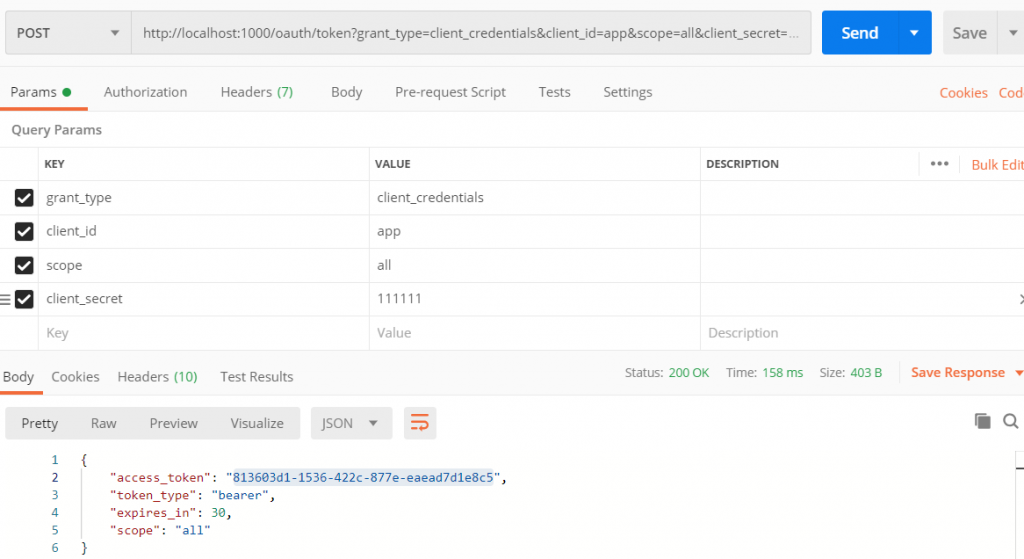
根据token访问后台
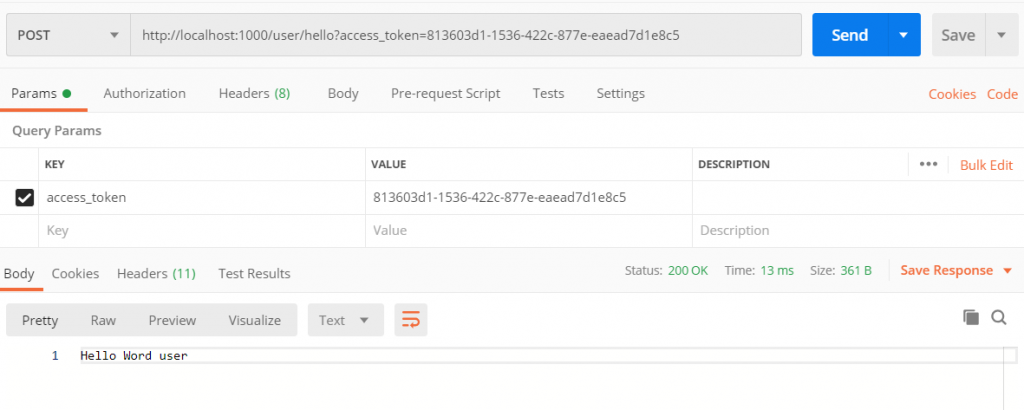
注意:以上两次访问后台对应的token授权方式肯定要和上面配置的一致,不然就会报错